這章在講設計 API 的 best practice。
Designing for Real-Life Use Cases
設計 API 時要以特定的、實際的 use case 進行決策,不要空想。
千萬不要公開內部基礎架構,把焦點放在與外部開發者(或 API 使用者)與 API 的互動體驗上。
藉由選擇特定的工作流程或 use case,你可以把重點放在一項設計上,並測試它是否可以幫助你的使用者。
在 brainstorming 階段去想「如果怎樣的話…」是有幫助的,可是到了設計階段,太多的「如果怎樣的話」的想像,反而會讓設計失焦,所以針對特定的 use case 是比較好的。
Designing for a Great Developer Experience
提供開發者好的開發體驗。
讓 API 能更快、更容易上手
文件可以幫開發者上手,有 tutorial 跟 getting started guide 也很好。
也可以提供線上互動式文件、線上的 sandbox 來讓 developer 可以實際測試。
提供 SDK 也可以幫助 developer 使用 API。
最後是應該要讓 developer 不需要登入或註冊就能使用 API。如果一定得要註冊,要盡可能減少註冊需要的資料。如果 API 使用 OAuth 保護,那麼應該要能讓 developer 在 UI 中產生 token 以使用 API。
維持一致性
像是 entry nmae、request 參數、response 等等,應該要維持一致性,讓 developer 即使不看文件也能猜到部份的 API。
在漸進修改的過程中,盡量與既有的設計模式保持一致,對使用者來說是最好的做法。
不要讓同樣的東西使用不同的名稱。
一致性很重要的原因是它可以減少試著了解你的 API 的 developer 的認知負擔(cognitive load)
Make Troubleshooting Easy
藉由回傳有意義的 error 跟 building tool 做到這點。
設計 API 時應該有系統的組織跟分類錯誤以及他們的回傳方式來方便 developer 排除問題。
有意義的 error 容易了解、明確而且可以讓人採取行動。它們可以協助 developer 了解問題並處理它。提供這些 error 的 detail 可以帶來較佳的使用者體驗。
machine-readable 的 error code 字串可以讓 developer 以程式處理錯誤。
除了 machine-readable 的 error code 之外,也可以加入 human-readable 的敘述,來讓 developer 更了解發生了什麼問題。
error 要講出具體錯誤的原因,像是「token 因為被撤銷而造成驗證失敗」,用 token_revoked 比 invalid_auth 好。
幫 error 分類
將 API request 過程(從 request 開始,到 architecture 的各種 service 邊界)的各種 high-level error 分門別類,例如:
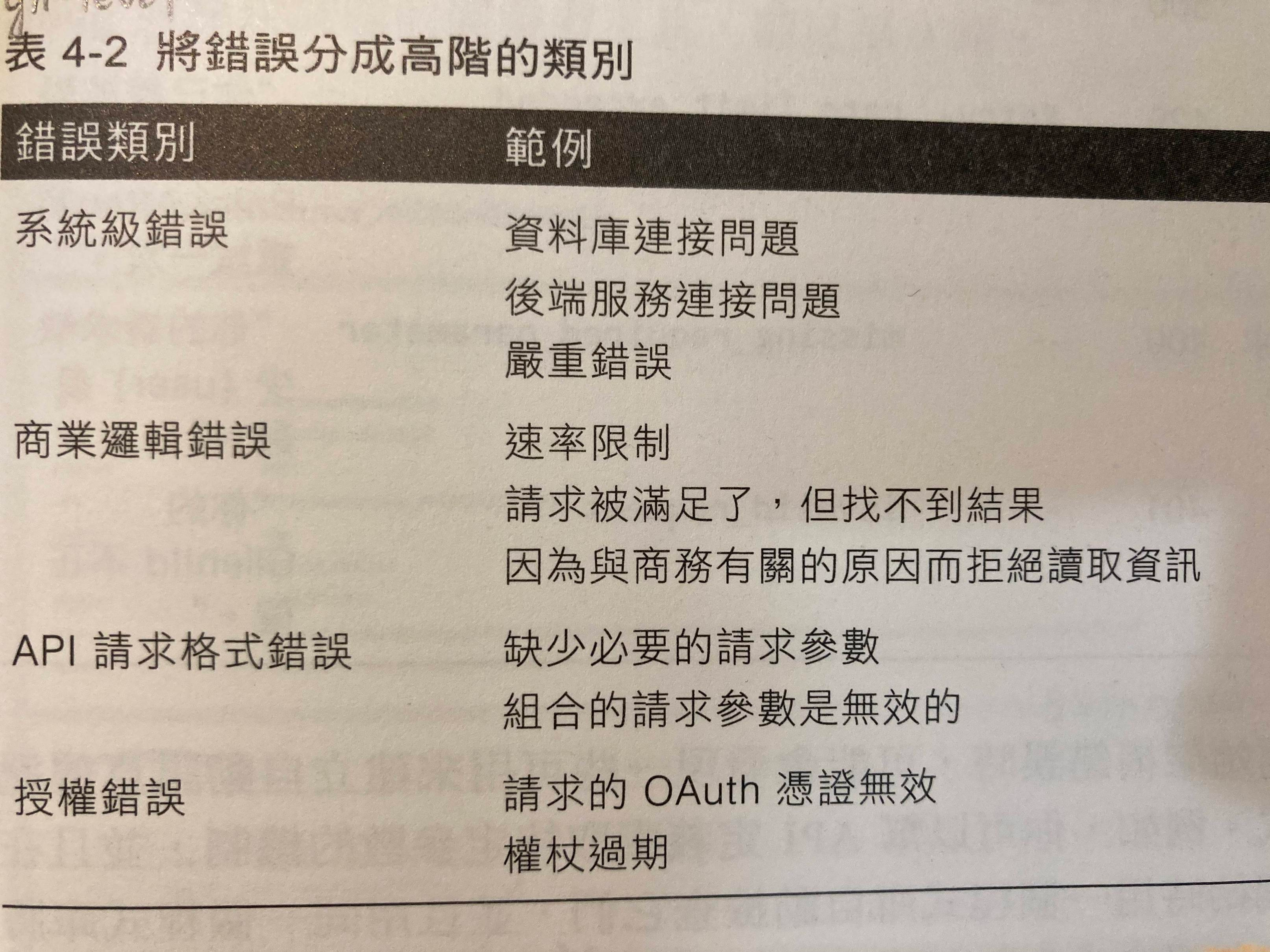
按照程式碼路徑將 error 分類後,要考慮對這些 error 而言,採取哪個 level 的 communication 是有意義的。一種方式是在 response payload 中放入 HTTP status code 跟 header,以及 machine-readable 的 code 或更詳細的 human-readable 的錯誤訊息。
大部分情況下,要盡量具體的說明來讓 developer 採取正確的後續動作。但有些時候,尤其跟安全有關的時候,可能要回傳比較籠統的資訊,不然原始訊息可能會透漏如資料庫 connection 資訊等會引發安全問題的資訊。
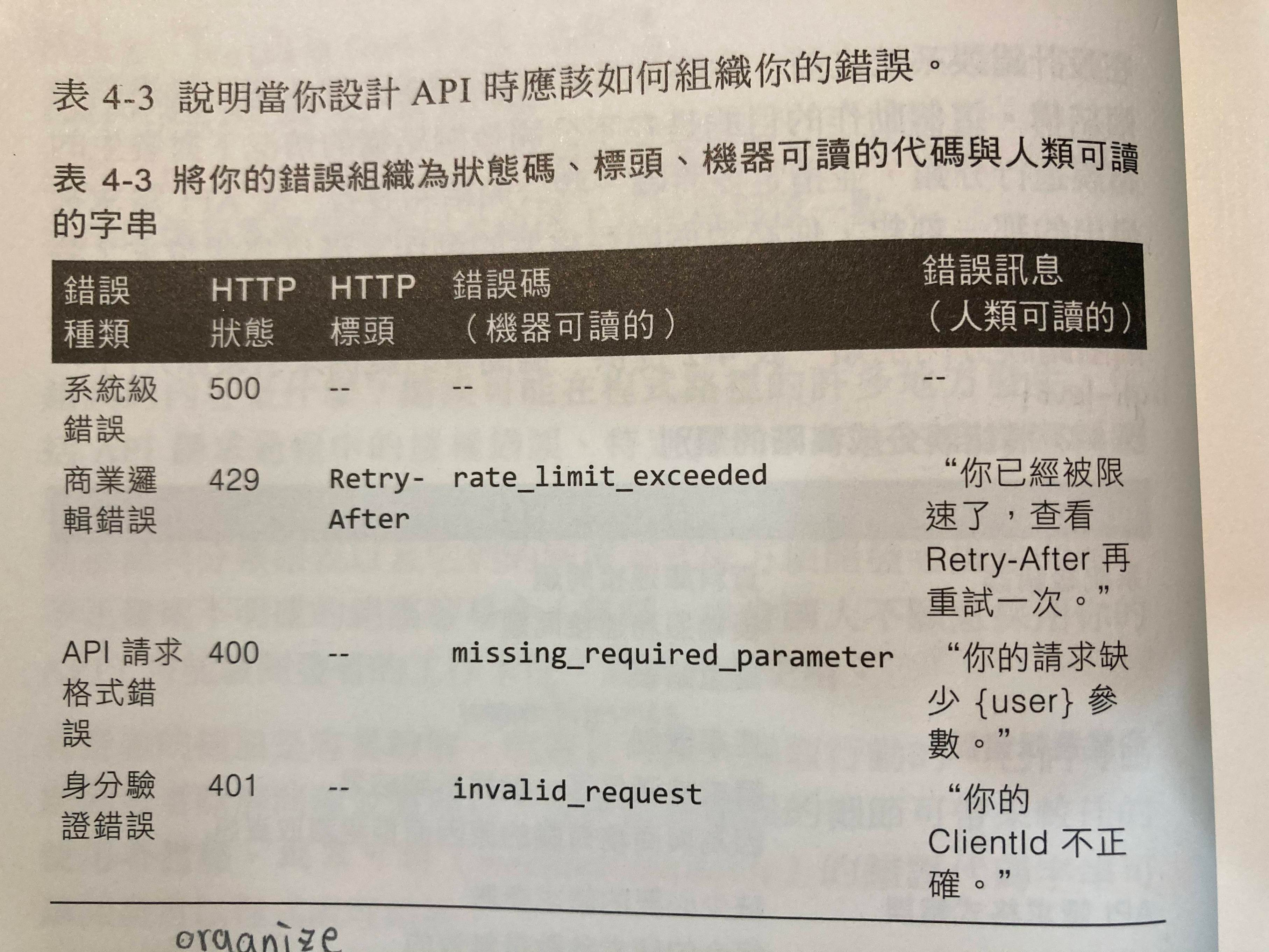
在程式結構上,可以用一套相同的 library 檢查 request 並將 error format 成相同的格式來 response。
將 error 文件化,像是寫在 API 文件中。
與 HTTP API 錯誤與問題相關可以參考 RFC 7807。
log HTTP status、error、error 的頻率以及其他 request metadata,好方便在內部或外部進行 debug 或處理問題。
建立 dashboard 來協助 developer 分析 API request 的 metadata,例如可以統計最常用的 API entry、找出沒被用過的 API 參數、分類常見錯誤等等。
log 跟 dashboard 都有很多現成的工具。
Make Your API Extensible
要擬定 API 的發展策略,讓 API 是可擴展的(extensible)。
API 應該提供可開啟新工作流程的基本元素,而非只是對映你的 app 的工作流程。API 的建立方式決定了 API 的使用者可用他來做什麼事情。如果你提供太低階的操作,可能會讓整合者負擔太多工作。如果你提供太高階的操作,可能會讓大多數的整合只是對應你自己的 app 所作的工作而已。為了實踐創新,你必須找到適當的平衡點,讓使用者能夠啟動不屬於你的 app 或 API 本身的工作流程。
在前後端分離的架構下,要區分內部使用跟對外公開的 API。兩者對於要提供什麼樣的 API 會有不同的考量。
extensible 的其中一個部份是確保 top partner 有提供 feedback 的機會。要設法 release 某些功能給 top partner 用用看,讓他們給予 feedback。
在想要用版本管理 API 時,如果早期就加入版本管理系統會比較容易,越晚加入越難實作。版本管理系統的好處在於它可以讓你用新版本進行 breaking changes,同時又能維持就版本的回溯相容性(backward compatibility)。breaking changes 就是會讓之前使用你的 API 可以正常運作的 app 無法繼續運作的 changes。
不過,維護版本是需要成本的。如果很多年沒有能力支援舊版本,或者認為 API 不太需要變動,那麼可以不用版本,改用 additive(附加式)變動策略,在單一、穩定的版本中維持 backward compatibility。
如果預計在「未來任何時刻」都有可能出現 breaking changes 與更新,最好建立版本管理系統。在一開始就建立版本管理系統需要付出的成本比之後迫切需要它的時候才加入低得多。